Phase 0 - Week 5 The Cooking Class
Friday, May 29, 2015

Since Ruby is an object oriented programming language, it includes Classes and Objects. The best description that I have found for Classes is that they are the blueprints that define how new Objects are created. The Class contains variables and methods that are standard to all Objects that are created as instances of that Class. As an example, today we will talk about the Cooking Class. This new Class will be the blueprint that we will use to create individual Recipe Objects that can be used to work with recipes for home cooks. Below is a visual representation of our new Cooking Class and all of the Instance Variables and Instance Methods that it carries with it.
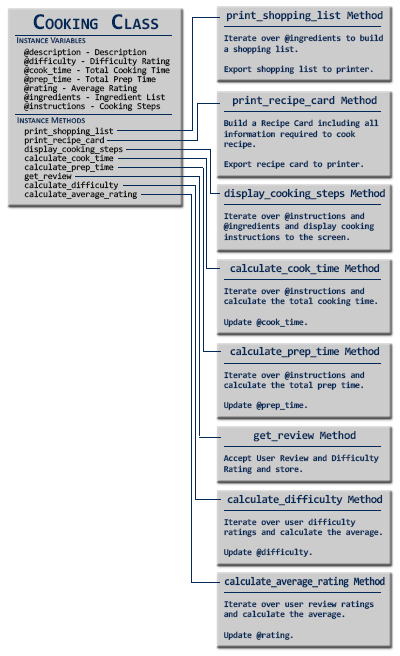
Inside of our new Cooking Class, there are 7 Instance Variables all noted with an @ symbol in front of the variable name. Instance variables are avaiable to all methods contained within the Class without being passed to the method. In our Cooking Class, we have Instance Variables for:
Variable Name | Purpose |
---|---|
@description | Contains a Description and/or Overview of the Recipe |
@difficulty | Stores the Calculated User Difficulty Rating |
@cook_time | Stores the Calulated Cook Time |
@prep_time | Stores the Calulated Prep Time |
@rating | Stores the Calulated Average User Rating |
@ingredients | Stores List of Ingredients passed to the Class |
@instructions | Stores the Step by Step Instructions passed to the Class |
In the initialization of the new Class, some of the Instance Variables listed above are assigned variables that are passed are parameters that are passed when the new Class is created and the remainder are initialized to default values and changed by the Instance Methods contained in the Class.
In addition to the Instance Variables, this Class also contains 8 Instance Methods that perform various functions specific to every new object that is created as an instance of the Cooking Class. For the purpose of this Blog, the specific code for each Instance Method is not critical; however, the Pseudocode for each Method is listed.
Below is an example of the code that is required to create our new Cooking Class and initialize each of the Instance Variables. To complete our new Cooking Class, we would follow this block of code with the definitions for each of the Instance Methods and all of the code required to perform the tasks for each.
Defining The Cooking Class
Once we have our new Cooking Class defined, we can then use it as the blueprint that will define how each of the new Recipe Objects will look. Each new Recipe Object that we create will be instances of the Cooking Class. It is easy to understand how Classes streamline our code and significantly reduce the amount of code that is required to create new objects.